Macにgoをインストールして、簡単な動作確認をします。
GOROOT・GOPATHの役割、goコマンド(env, get, build, run, mod)、GoLandの使い方について確認します。
インストール
Goのバイナリは下記ページからダウンロードできます。
Downloads – The Go Programming Language
ここでは、Homebrewを利用して、Macにgoをインストールします。
brew install go
インストール後、goコマンドが実行できるか確認します。
$ go
Go is a tool for managing Go source code.
Usage:
go <command> [arguments]
The commands are:
bug start a bug report
build compile packages and dependencies
clean remove object files and cached files
doc show documentation for package or symbol
env print Go environment information
fix update packages to use new APIs
fmt gofmt (reformat) package sources
generate generate Go files by processing source
get add dependencies to current module and install them
install compile and install packages and dependencies
list list packages or modules
mod module maintenance
run compile and run Go program
test test packages
tool run specified go tool
version print Go version
vet report likely mistakes in packages
Use "go help <command>" for more information about a command.
Additional help topics:
buildconstraint build constraints
buildmode build modes
c calling between Go and C
cache build and test caching
environment environment variables
filetype file types
go.mod the go.mod file
gopath GOPATH environment variable
gopath-get legacy GOPATH go get
goproxy module proxy protocol
importpath import path syntax
modules modules, module versions, and more
module-get module-aware go get
module-auth module authentication using go.sum
module-private module configuration for non-public modules
packages package lists and patterns
testflag testing flags
testfunc testing functions
Use "go help <topic>" for more information about that topic.
環境変数( go env )
go env
を実行するとgoの環境変数について確認できます。
GOROOT
Goがインストールされた場所は、GOROOT
で確認できます。
$ go env | grep GOROOT
GOROOT="/usr/local/Cellar/go/1.15.6/libexec"
$ ls -l /usr/local/Cellar/go/1.15.6/libexec
total 248
-rw-r--r-- 1 xxx staff 1339 Dec 4 02:32 CONTRIBUTING.md
-rw-r--r-- 1 xxx staff 95475 Dec 4 02:32 CONTRIBUTORS
-rw-r--r-- 1 xxx staff 1303 Dec 4 02:32 PATENTS
-rw-r--r-- 1 xxx staff 397 Dec 4 02:32 SECURITY.md
-rw-r--r-- 1 xxx staff 8 Dec 4 02:32 VERSION
drwxr-xr-x 21 xxx staff 672 Dec 4 02:32 api
drwxr-xr-x 5 xxx staff 160 Dec 4 02:32 bin
drwxr-xr-x 45 xxx staff 1440 Dec 4 02:32 doc
-rw-r--r-- 1 xxx staff 5686 Dec 4 02:32 favicon.ico
drwxr-xr-x 3 xxx staff 96 Dec 4 02:32 lib
drwxr-xr-x 14 xxx staff 448 Dec 4 02:32 misc
drwxr-xr-x 6 xxx staff 192 Dec 4 02:32 pkg
-rw-r--r-- 1 xxx staff 26 Dec 4 02:32 robots.txt
drwxr-xr-x 69 xxx staff 2208 Dec 4 02:32 src
drwxr-xr-x 328 xxx staff 10496 Dec 4 02:32 test
goコマンドなどは、binフォルダ配下に格納されています。
$ ls -l /usr/local/Cellar/go/1.15.6/libexec/bin/
total 65864
-rwxr-xr-x 1 xxx staff 14125324 Dec 4 02:32 go
-rwxr-xr-x 1 xxx staff 16145836 Dec 4 02:32 godoc
-rwxr-xr-x 1 xxx staff 3447872 Dec 4 02:32 gofmt
GOPATH
外部から取得したパッケージの格納先を決める際、GOPATHの影響を受けます。
$ go env | grep GOPATH
GOPATH="/Users/xxx/go"
例として、echoをダウンロードしてみます。
$ go get github.com/labstack/echo/...
$ tree -L 1 /Users/xxx/go
/Users/xxx/go
├── pkg
└── src
src配下に echoパッケージ と echoの依存パッケージ のソースコードが格納されています。
$ tree -L 3 /Users/xxx/go/src
/Users/xxx/go/src
├── github.com
│ ├── dgrijalva
│ │ └── jwt-go
│ ├── labstack
│ │ ├── echo
│ │ └── gommon
│ ├── mattn
│ │ ├── go-colorable
│ │ └── go-isatty
│ └── valyala
│ └── fasttemplate
└── golang.org
└── x
├── crypto
├── net
├── sys
├── text
└── time
pkg配下にビルド後のパッケージオブジェクトが格納されています。
$ tree -L 4 /Users/xxx/go/pkg
/Users/xxx/go/pkg
└── darwin_amd64
└── github.com
└── labstack
├── echo
└── echo.a
GoLandの設定
[GoLand] - [Preferences]
を開きます。
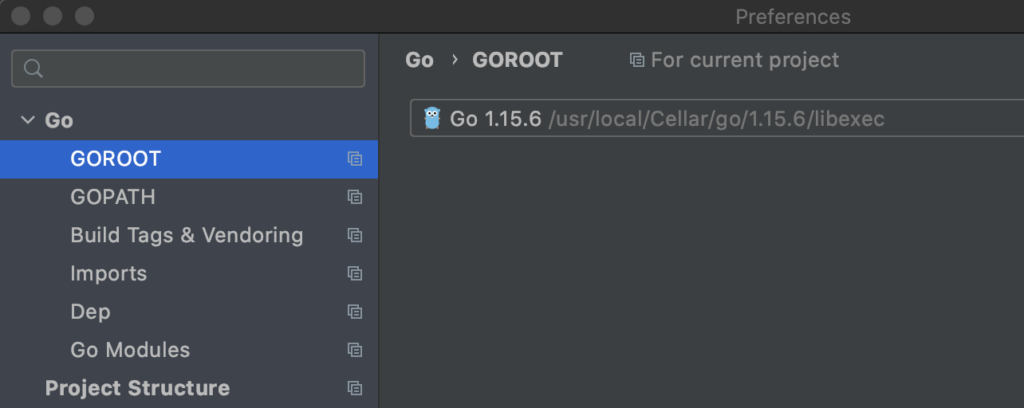
GOROOTの設定画面です。
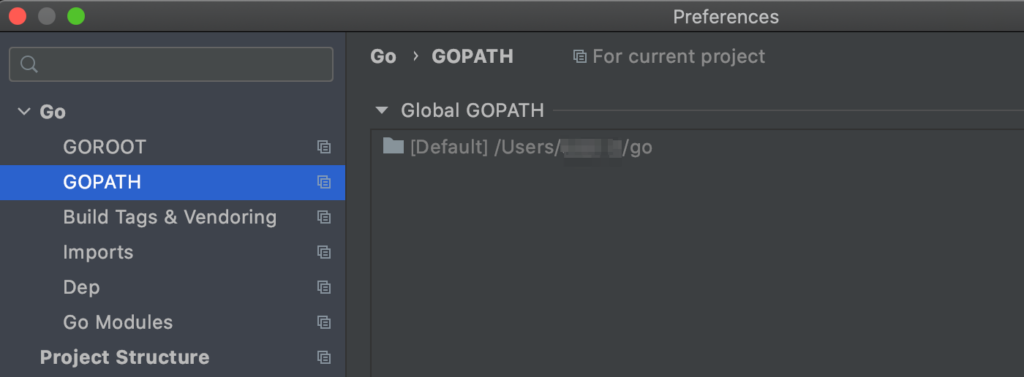
GOPATHの設定画面です。
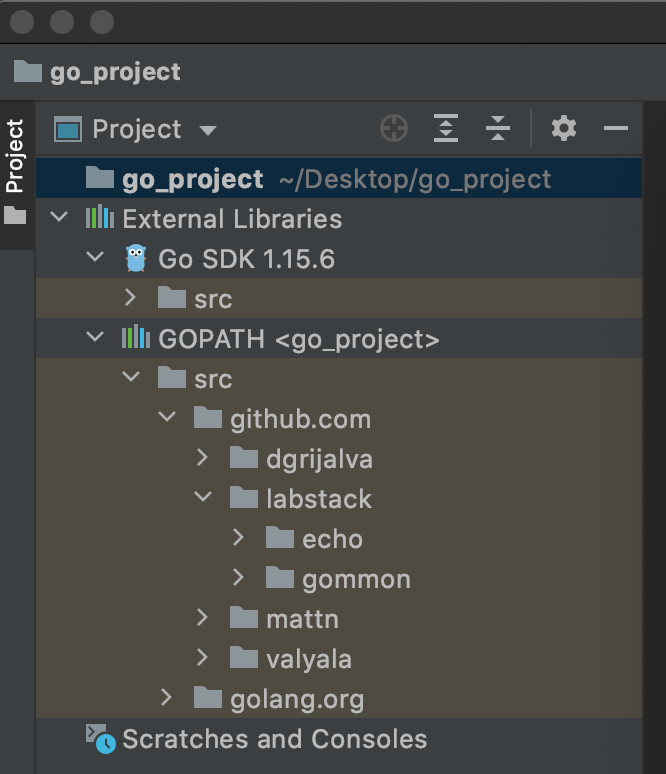
正しく設定されていれば、GO SDKとGOPATHについて表示されます。
Hello Worldの確認
main.goファイルを作り、以下処理を記述します。
package main
import "fmt"
func main() {
fmt.Println("Hello world")
}
go build
go build
で実行バイナリを生成できます。
$ go build main.go
$ ls -l
total 4200
-rwxr-xr-x 1 xxx staff 2142872 Dec 4 02:32 main
-rw-r--r-- 1 xxx staff 73 Dec 4 02:32 main.go
生成された実行バイナリを起動してみます。
$ ./main
Hello world
go run
go run
を利用するとビルドと実行を同時に行えます。
$ go run main.go
Hello world
GoLandで実行・デバッグ
まずは通常実行を確認します。
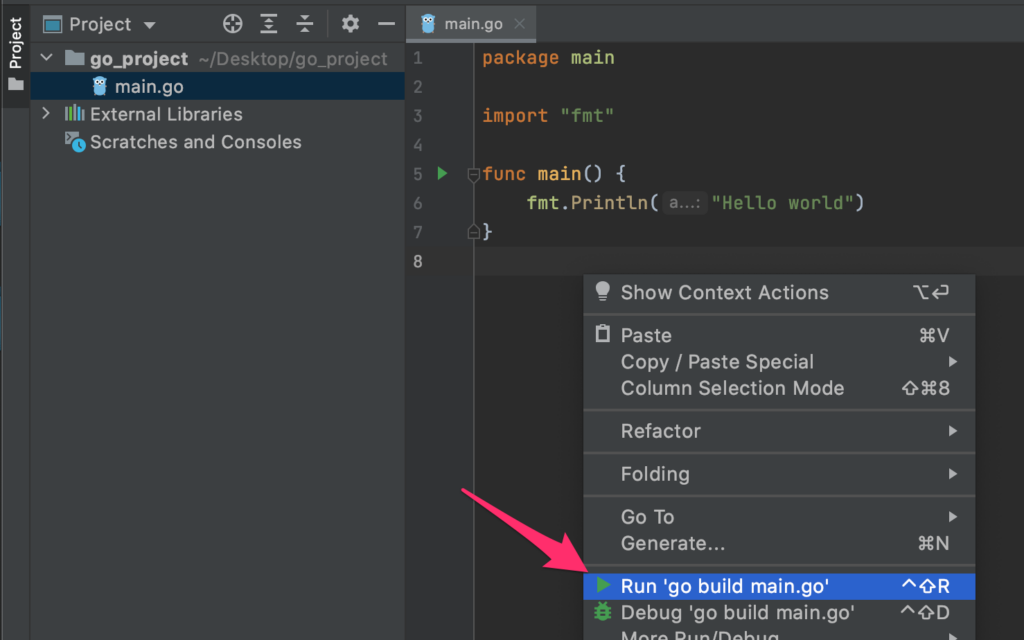
矢印箇所をクリックすると実行できます。
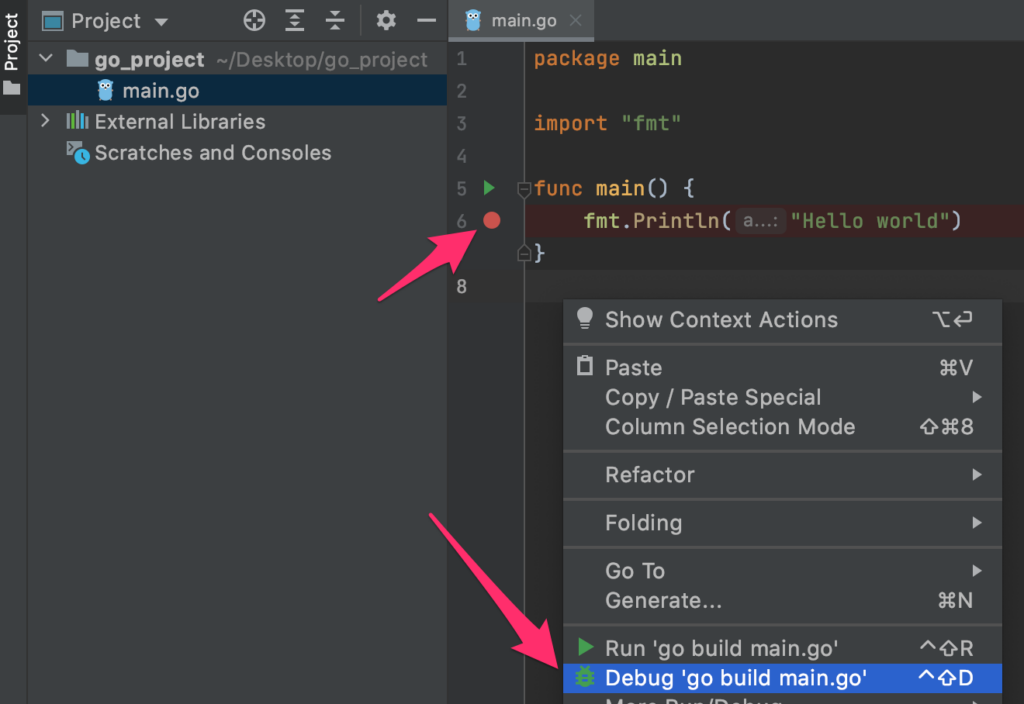
ブレイクポイントを入れた状態でデバッグを実行します。
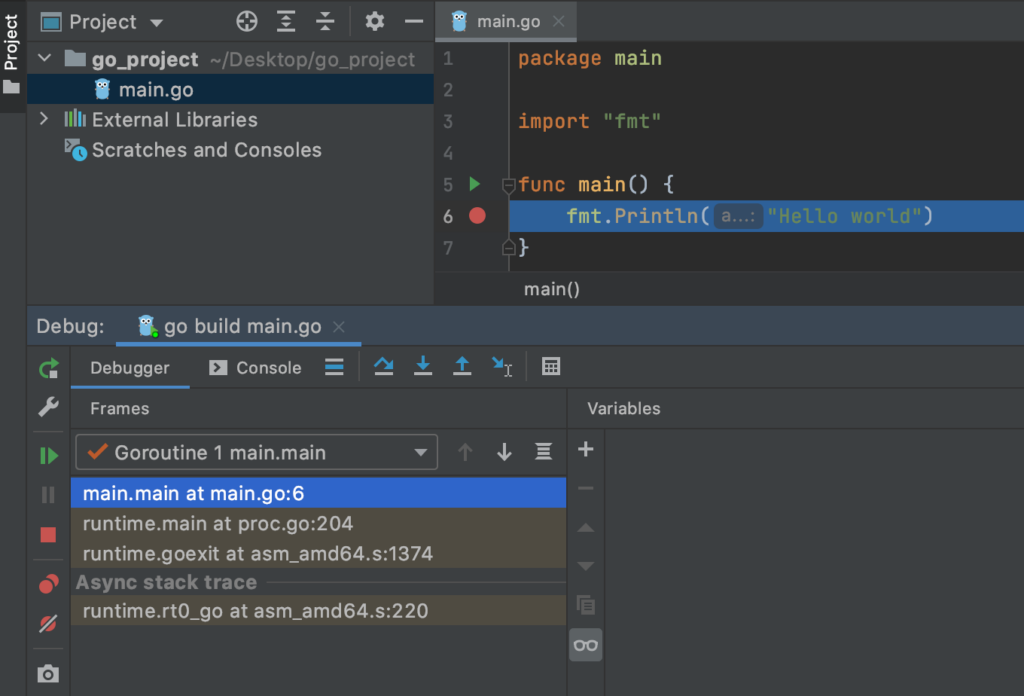
ブレイクポイントで処理が止まりました。
go modによるモジュール管理
Go言語の 1.11以上 で go mod
が使えるようになりました。
依存モジュールを go.modファイル と go.sumファイル で管理できます。
go mod
では以下のサブコマンドを利用できます。
$ go help mod
Go mod provides access to operations on modules.
Note that support for modules is built into all the go commands,
not just 'go mod'. For example, day-to-day adding, removing, upgrading,
and downgrading of dependencies should be done using 'go get'.
See 'go help modules' for an overview of module functionality.
Usage:
go mod <command> [arguments]
The commands are:
download download modules to local cache
edit edit go.mod from tools or scripts
graph print module requirement graph
init initialize new module in current directory
tidy add missing and remove unused modules
vendor make vendored copy of dependencies
verify verify dependencies have expected content
why explain why packages or modules are needed
Use "go help mod <command>" for more information about a command.
go mod init
go mod init
で初期化します。
$ go mod init example.com/xxx
go.modファイルが作成されました。
$ cat go.mod
module example.com/xxx
go 1.15
go mod tidy
main.goに以下処理を記述します。
package main
import (
"net/http"
"github.com/labstack/echo/v4"
)
func main() {
// Echo instance
e := echo.New()
// Routes
e.GET("/", hello)
// Start server
e.Logger.Fatal(e.Start(":1323"))
}
// Handler
func hello(c echo.Context) error {
return c.String(http.StatusOK, "Hello, World!")
}
echoパッケージを利用しています。
go mod tidy
を利用すると、依存モジュールを自動インストールして、使われていない依存モジュールを削除してくれます。
$ go mod tidy
go: finding module for package github.com/labstack/echo/v4
go: downloading github.com/labstack/echo v1.4.4
go: downloading github.com/labstack/echo/v4 v4.1.17
go: downloading github.com/labstack/echo v3.3.10+incompatible
go: found github.com/labstack/echo/v4 in github.com/labstack/echo/v4 v4.1.17
go: downloading github.com/labstack/gommon v0.3.0
(省略)
module example.com/xxx
go 1.15
+ require github.com/labstack/echo/v4 v4.1.17
GoLandでModulesを有効にする
[GoLand] – [Preferences] を開きます。
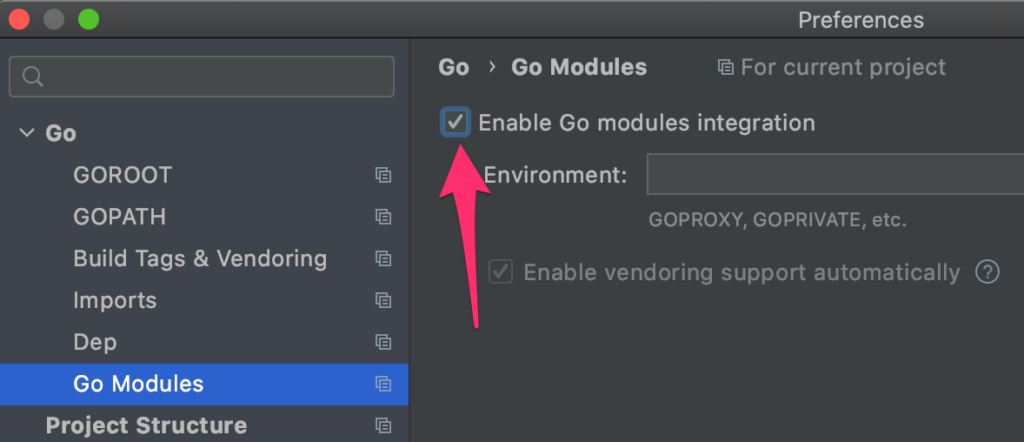
Enable Go modules integrationにチェックを入れます。
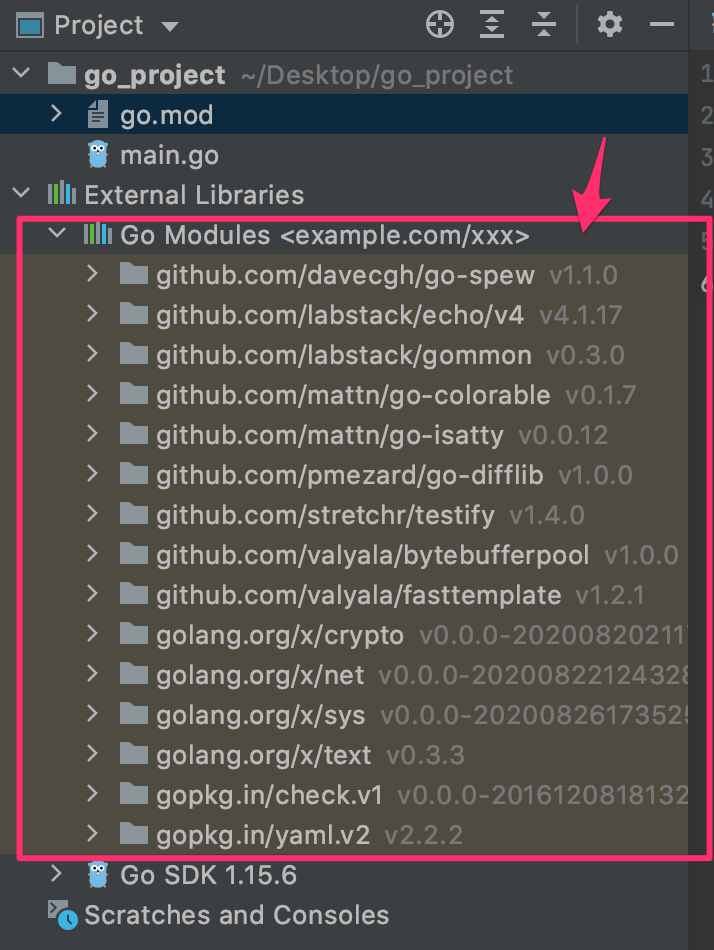
Go Modulesが認識されました。
go getとgo install
goの1.16から、go get
と go install
の使い分けがわかりやすくなりました。
go get
は、go.modの編集に利用します。go install
は、ツールのグローバルインストールに利用します。
go install の使い方
以下の形式で利用できます。
go install example.com/cmd@v1.0.0
バージョン指定は必須です。最新のバージョンを指定したい場合 @latest
をつけます。
GOPATH
が設定されている場合、$GOPATH/bin
配下に実行バイナリがインストールされます。
$ go install github.com/tsenart/vegeta@latest
go: downloading github.com/tsenart/vegeta v1.2.0
(略)
$
$
$ ls $GOPATH/bin
vegeta