ESLintとPrettierを活用すると、「コード実行前の静的検証によるバグ検出」「コーディングスタイルの統一」といったメリットを得ることができます。ここでは、ESLintとPrettierの基本的な利用法を確認します。
ESLint
インストール
npm i eslint --save-dev
今回、typescriptを利用したプロジェクトにESLintを導入するので typescript
をインストールしておきます。
npm i typescript --save-dev
eslint init
以下コマンドでESLintの設定を簡単にすることができます。
./node_modules/.bin/eslint --init
$ ./node_modules/.bin/eslint --init
? How would you like to use ESLint? To check syntax and find problems
? What type of modules does your project use? JavaScript modules (import/export)
? Which framework does your project use? None of these
? Does your project use TypeScript? Yes
? Where does your code run? (Press <space> to select, <a> to toggle all, <i> to invert selection)Browser
? What format do you want your config file to be in? JavaScript
The config that you've selected requires the following dependencies:
@typescript-eslint/eslint-plugin@latest @typescript-eslint/parser@latest
? Would you like to install them now with npm? Yes
Installing @typescript-eslint/eslint-plugin@latest, @typescript-eslint/parser@latest
npm WARN eslint-prettier-test@1.0.0 No description
npm WARN eslint-prettier-test@1.0.0 No repository field.
+ @typescript-eslint/parser@2.3.2
+ @typescript-eslint/eslint-plugin@2.3.2
added 8 packages from 9 contributors in 7.546s
Successfully created .eslintrc.js file in /tmp/eslint-prettier-test
.eslintrc.js
ファイルが生成されました。以下の内容が記述されていました。
module.exports = {
"env": {
"browser": true,
"es6": true
},
"extends": [
"eslint:recommended",
"plugin:@typescript-eslint/eslint-recommended"
],
"globals": {
"Atomics": "readonly",
"SharedArrayBuffer": "readonly"
},
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaVersion": 2018,
"sourceType": "module"
},
"plugins": [
"@typescript-eslint"
],
"rules": {
}
};
実行例
.eslintrc.js
に以下ルールを追加します。
module.exports = {
(省略)
"rules": {
'indent': ['error', 2],
}
};
ESLintの実行例を示します。app.ts
というサンプルファイルを検証してみます。
$ cat -n app.ts
1 function greeter(person: string)
2 {
3 return 'Hello, ' + person
4 }
5
6 let user = 'わくわくBank.'
7
8 console.log(greeter(user))
$
$
$ ./node_modules/.bin/eslint app.ts
/tmp/eslint-prettier-test/app.ts
3:1 error Expected indentation of 2 spaces but found 4 indent
✖ 1 problem (1 error, 0 warnings)
1 error and 0 warnings potentially fixable with the `--fix` option.
indentルール違反が見つかりました。
以下のように、--fixオプション
で自動修正してくれます。
$ ./node_modules/.bin/eslint app.ts --fix
$
$ cat -n app.ts
1 function greeter(person: string)
2 {
3 return 'Hello, ' + person
4 }
5
6 let user = 'わくわくBank.'
7
8 console.log(greeter(user))
設定情報記述場所
ESLintの設定情報を記述する場所は、以下の方法から選択できます。
.eslintrc
ファイルを作成して記述.eslintrc.js
ファイルを作成して記述.eslintrc.json
ファイルを作成して記述.eslintrc.yml
ファイルを作成して記述package.json
にeslintConfig
フィールドを作って記述
チェック対象外にする
( .eslintignore )
.eslintignore
ファイルを作成して、ESLintのチェック対象外にしたいファイルパスを記述します。
ルールの設定
以下、ルール設定に関する大まかな構成です(.eslintrc.js
)。
module.exports = {
extends: [
// 「ルールの追加」や「ルールのオプションの拡張」をすることができます。
],
plugins: [
// サードパーティのルールを指定できます。
// パッケージインストールが必要です。
],
rules: {
// ルールの上書きや追加ルールの設定を行います。
}
}
以下、ルールの指定方法です。
module.exports = {
(省略)
rules: {
// オプションなしルール
'ルール名': 'off', // ルールをオフにする
'ルール名': 'warn', // ルール違反の場合、警告とする
'ルール名': 'error', // ルール違反の場合、エラーとする
// オプションありルール
// オプション有りの場合、配列指定します。
// 以下、通常オプションのみの例です。
'semi': ['error', 'always'], // セミコロンを常に使う。
'semi': ['error', 'never'], // セミコロンを禁止する。
'quotes': ['error', 'single'], // 文字列指定をシングルクォートにする
// 以下、通常オプションとオブジェクトオプションをもつ例です。
'indent': ['error', 2, { 'SwitchCase': 1 }] // スペース2つをインデントにする。
// switchステートメントのcase句のインデントレベルを強制する。
}
}
- ルールに対しての動作を指定しています
off
warn
error
- ルールにオプションがあれば、必要に応じてオプションも指定します。
参考
Prettier
ESLintでは対応できないコード整形をすることができます。
インストール
npm i prettier --save-dev
設定
.prettierrc.js
という設定ファイルを作成します。例として、以下内容を記述しました。
module.exports = {
trailingComma: "es5",
semi: true,
singleQuote: true
};
実行例
どのように整形されるのか確認します。
$ cat app.ts
function greeter(person: string)
{
return "Hello, " + person
}
const user = "わくわくBank."
console.log(greeter(user))
const obj = {
a: 1,
b: 2,
c: 3
}
console.log(obj)
$
$
$
$
$ node_modules/.bin/prettier app.ts
function greeter(person: string) {
return 'Hello, ' + person;
}
const user = 'わくわくBank.';
console.log(greeter(user));
const obj = {
a: 1,
b: 2,
c: 3,
};
console.log(obj);
今回のPrettier設定によって、以下の整形が行われました。
- objの最後要素にカンマが追加される
- セミコロンが追加される
- シングルクォーテーションで囲まれる
整形された状態で上書きするには、--writeオプション
を利用します。
$ node_modules/.bin/prettier --write app.ts
ESLintから利用
パッケージを追加でインストールします。
npm i eslint-config-prettier eslint-plugin-prettier --save-dev
.eslintrc.js
の extendsプロパティ
に plugin:prettier/recommended
を追記します。
module.exports = {
(省略)
"extends": [
(省略)
"plugin:prettier/recommended"
],
(省略)
};
以下のように、ESLintを実行するとPrettierも実行されるようになりました。
$ cat -n app.ts
1 function greeter(person: string)
2 {
3 return "Hello, " + person
4 }
5
6 const user = "わくわくBank."
7 console.log(greeter(user))
8
9 const obj = {
10 a: 1,
11 b: 2,
12 c: 3
13 }
14 console.log(obj)
$
$
$
$ ./node_modules/.bin/eslint app.ts
/tmp/eslint-prettier-test/app.ts
1:33 error Replace `⏎` with `·` prettier/prettier
3:10 error Replace `"Hello,·"·+·person` with `'Hello,·'·+·person;` prettier/prettier
6:14 error Replace `"わくわくBank."` with `'わくわくBank.';` prettier/prettier
7:27 error Insert `;` prettier/prettier
12:7 error Insert `,` prettier/prettier
13:2 error Insert `;` prettier/prettier
14:17 error Insert `;` prettier/prettier
✖ 7 problems (7 errors, 0 warnings)
7 errors and 0 warnings potentially fixable with the `--fix` option.
参考
WebStormでの利用
ESLintを有効にする
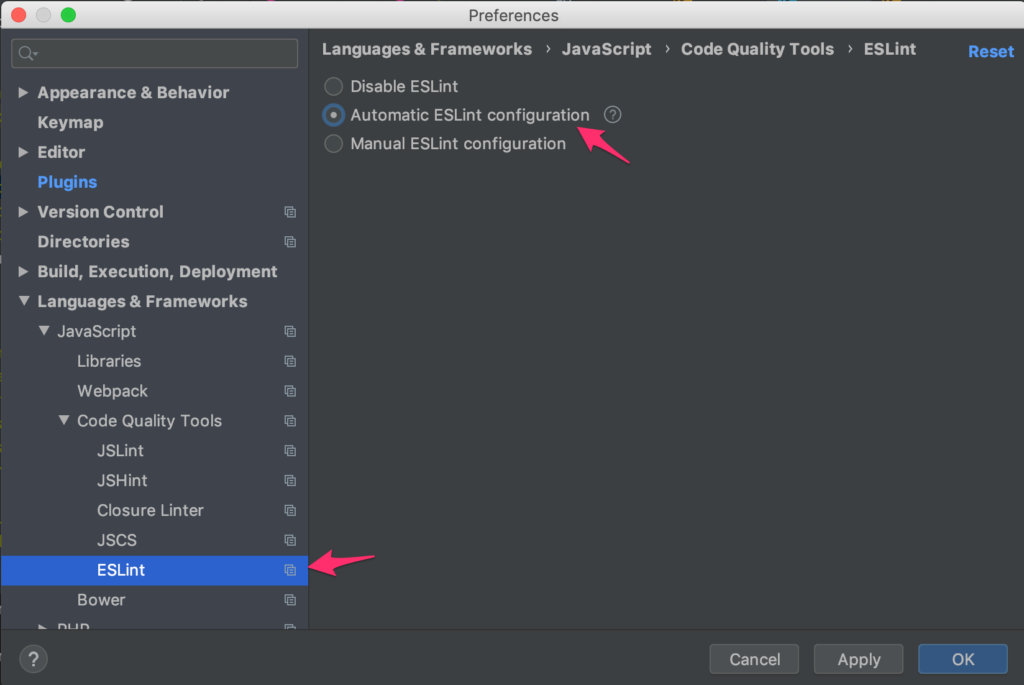
Languages & Frameworks
> JavaScript
> Code Quality Tools
> ESLint
を選択します。
Automatic ESLint configuration
にチェックを入れて反映させます。
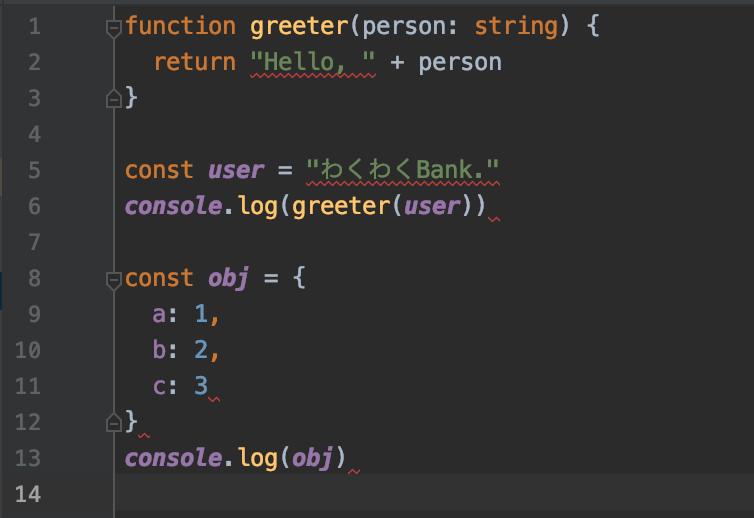
ESLintが有効化され、ルール違反箇所は赤波線で表示されるようになりました。
Keymapで整形作業の効率化
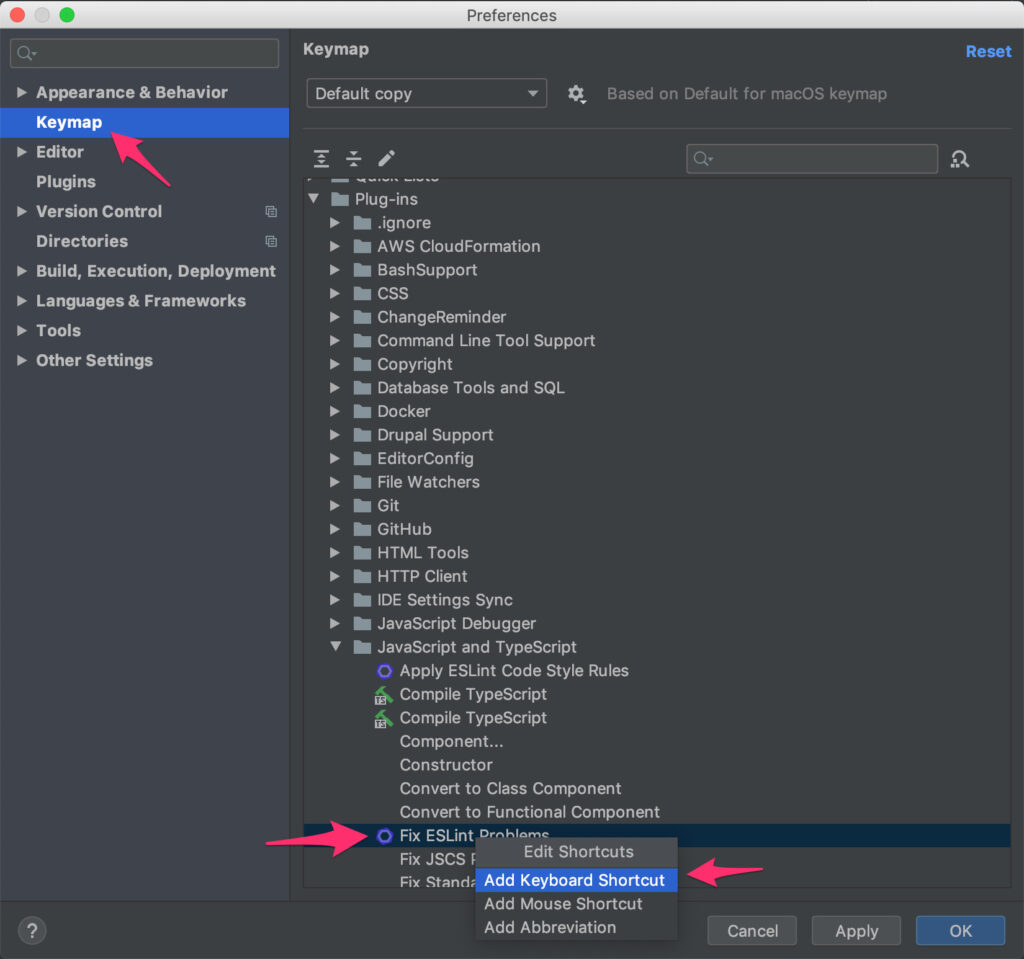
Keymap
> Plug-ins
> JavaScript and TypeScript
> Fix ESLint Problems
を選択して、ショートカットキーを割り当てます。
割り当てたショートカットキーを利用することで、ESLintの整形作業を手軽に行うことができます。