TypeScriptでは、Type Aliasesと呼ばれる機能を提供しています。Type Aliasesを利用すると型に別名を付けることができます。Interfaceと近い機能を持っていますが、挙動の違いも確認します。
目次
型に別名をつける
基本型である number
に Old
という別名をつけてみます。
type Old = number;
const old: Old = 37;
別名をつけることでコードの意味がわかりやすくなります。
implements
Classのimplementsに指定することもできます。
type TPerson = {
name: string;
old: number;
};
class Person implements TPerson {
name = 'yamada';
old = 18;
show(): string {
return `name: ${this.name} old: ${this.old}`;
}
}
const person = new Person();
console.log(person.show()); // name: yamada old: 18
拡張方法
Type Aliasesは交差型を利用して拡張
Interface
では 継承(extends)
を利用して拡張できます。
Type Aliases
では 交差型
を利用します。
type TPerson = {
name: string;
old: number;
};
type TPerson2 = TPerson & {
address: string;
};
Interfaceと比較
(同一の名前で再宣言)
Interfaceの場合、同一の名前で再宣言するとpropertyが追加されていきます。
interface Person {
name: string;
old: number;
}
interface Person {
address: string;
}
const echo = (person: Person) => {
console.log(person);
};
echo({ name: 'yamada', old: 18, address: 'xxx' });
Type Aliasesでは、同一名の宣言があると以下のようにエラーとなります。
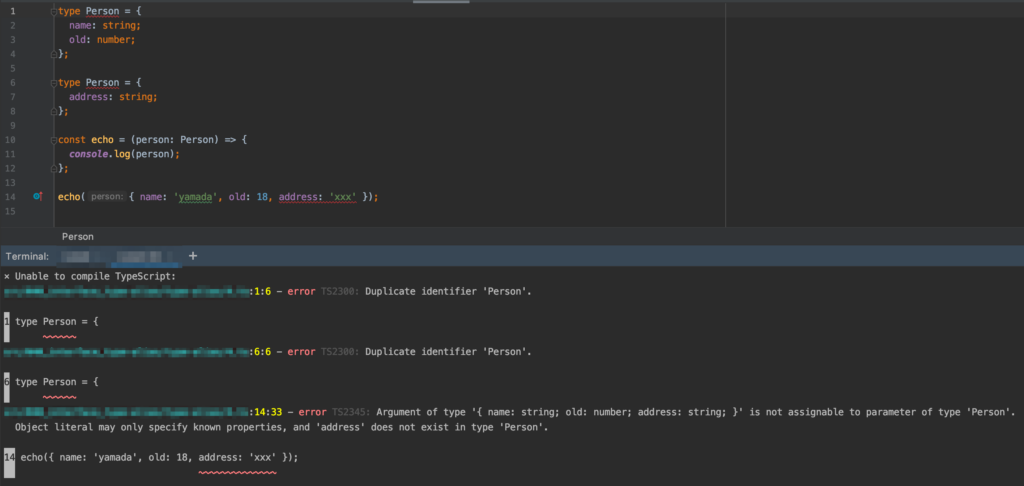
新しいプロパティを追加するには、新しく別名をつける必要があります。